Mastering MD5 Encode Decode in PHP for Web Development
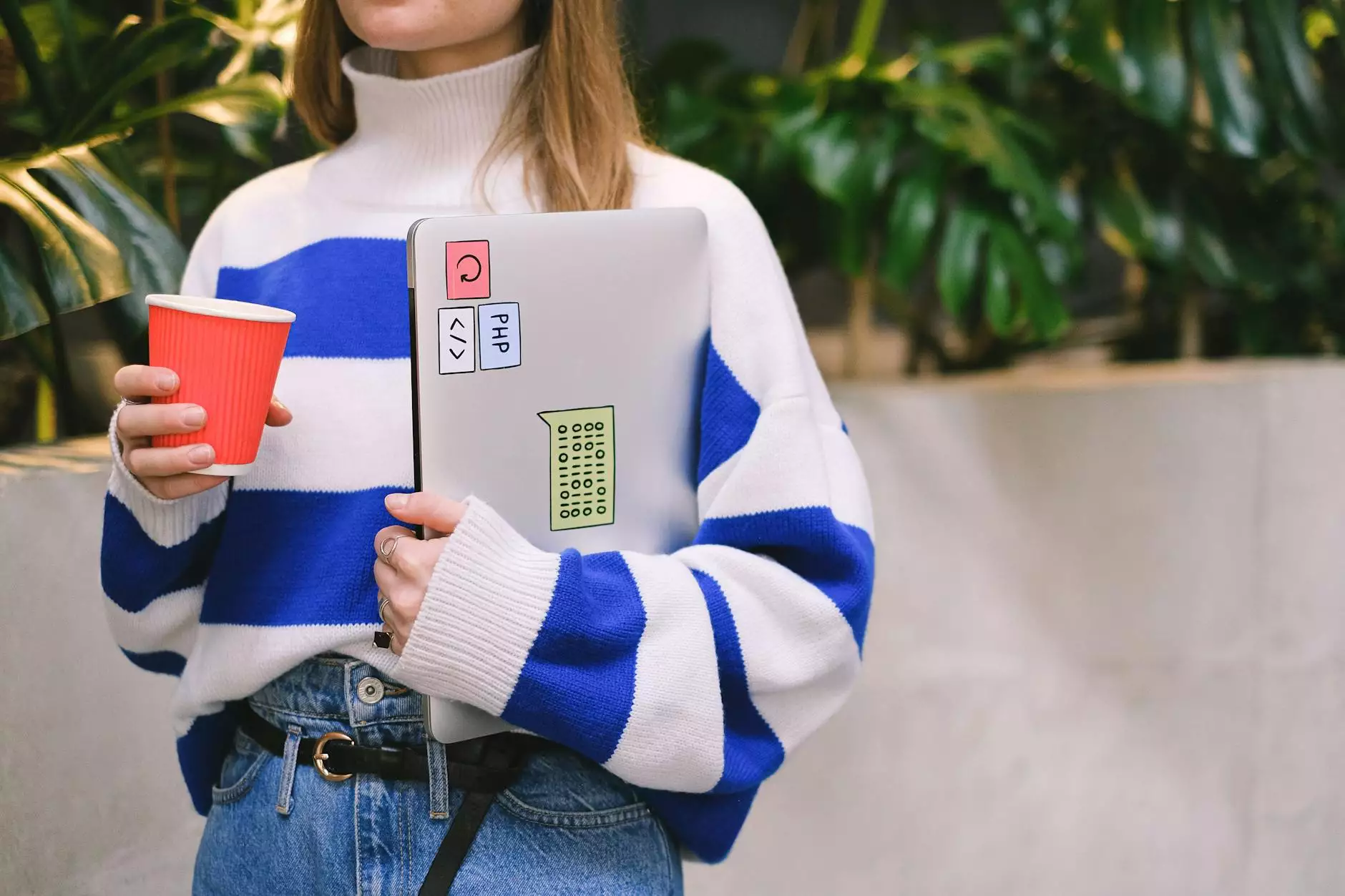
If you’re involved in web design or software development, understanding and utilizing MD5 encode decode in PHP is essential for creating robust, secure applications. This article delves into the depths of MD5 encoding and decoding using PHP, providing you with the necessary insights to effectively apply this hashing algorithm in your projects.
What is MD5?
MD5, or Message-Digest algorithm 5, is a widely used cryptographic hash function that produces a 128-bit hash value (32 hexadecimal characters). Initially designed for data integrity verification, it is now primarily used in various applications for password storage and data verification.
Why Use MD5 in PHP?
In the realm of web development, using MD5 has several benefits:
- Performance: MD5 is extremely fast in terms of execution speed.
- Compatibility: It is supported by nearly every programming language and platform, including PHP.
- Convenience: It’s easy to implement and requires minimal coding.
- Hashing for Passwords: MD5 is often employed for hashing passwords before storing them in databases to enhance security.
How to MD5 Encode in PHP
Encoding a string in MD5 format in PHP is straightforward. PHP provides a built-in function called md5() that generates the MD5 hash of a given string.
Basic Syntax
string md5 ( string $str [, bool $raw_output = false ] )Example Code:
Here’s a simple example of how to encode a string using MD5 in PHP:
Understanding MD5 Output
The output of the above code will be a string that looks like this:
MD5 encoded string: fc3ff98e404040258 JacquesYann 0a1364c06482c1a1e256a7cfa6946524How to MD5 Decode in PHP?
It’s important to note that MD5 is a one-way hashing algorithm, meaning it is not designed to be decoded. However, developers sometimes seek to match a hashed value against a known string, which involves encoding the string in question and comparing the hashes.
Simulating MD5 Decode
To simulate decoding, you essentially hash the original string and compare that hash with the one you possess. If they match, you have successfully “decoded” (or rather, validated) the string.
Security Considerations
While MD5 is fast and convenient, it is important to recognize its vulnerabilities:
- Collision Attacks: Two different inputs can produce the same MD5 hash.
- Rainbow Tables: Precomputed tables can quickly find original strings from their MD5 hashes.
- Not Suitable for Sensitive Data: MD5 should not be used for encryption of sensitive information like passwords. Instead, use stronger alternatives like bcrypt or SHA-256.
Alternatives to MD5
With the decline in MD5's use for secure applications, developers are transitioning to stronger hashing functions:
- SHA-1: Generally considered better than MD5, but still not secure for sensitive applications.
- SHA-256: A member of the SHA-2 family, providing a higher level of security.
- Bcrypt: Specifically designed for hashing passwords, offering adaptive performance.
Best Practices for Using MD5 in PHP
When dealing with MD5, remember these best practices:
- Use MD5 only for non-sensitive data: Reserve MD5 for applications where security is not a concern.
- Implement Salt: Always use a unique salt value when hashing passwords to protect against rainbow table attacks.
- Avoid using MD5 for passwords: Opt for stronger hashing mechanisms like SHA-256 or Bcrypt.
Conclusion
In summary, understanding how to use MD5 encode decode in PHP is a valuable skill for any web developer. While MD5 provides a fast and easy solution for generating hashes, it is crucial to recognize its limitations and vulnerabilities. Opt for more secure methods for sensitive data and keep abreast of best practices in security to ensure your applications remain robust and secure.
Explore More
For more resources on web development, software development, and security practices, visit semalt.tools. Stay updated with the latest trends and enhance your skills with comprehensive tutorials and articles tailored to your needs.
md5 encode decode php